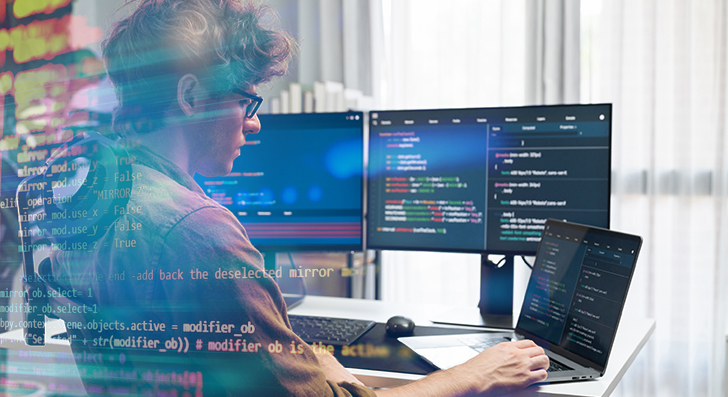
Scalability indicates your application can deal with growth—additional end users, more details, plus much more targeted traffic—without having breaking. As being a developer, setting up with scalability in mind will save time and pressure later. Listed here’s a clear and realistic guidebook that will help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability just isn't some thing you bolt on later—it ought to be component of your respective strategy from the start. Numerous purposes fail if they increase fast mainly because the original layout can’t handle the extra load. To be a developer, you should Imagine early about how your system will behave under pressure.
Start out by creating your architecture being adaptable. Stay away from monolithic codebases where by every thing is tightly linked. Alternatively, use modular structure or microservices. These patterns split your application into lesser, independent areas. Each individual module or services can scale on its own without affecting The entire process.
Also, think about your database from day one particular. Will it have to have to handle a million people or simply just a hundred? Choose the proper variety—relational or NoSQL—based upon how your details will grow. Strategy for sharding, indexing, and backups early, Even though you don’t have to have them yet.
An additional crucial level is to stop hardcoding assumptions. Don’t produce code that only will work less than present-day conditions. Think of what would transpire If the person foundation doubled tomorrow. Would your app crash? Would the database slow down?
Use style patterns that support scaling, like concept queues or occasion-driven methods. These help your application tackle extra requests without the need of having overloaded.
After you Make with scalability in your mind, you are not just planning for achievement—you're decreasing future problems. A very well-prepared program is simpler to keep up, adapt, and grow. It’s improved to get ready early than to rebuild later on.
Use the proper Databases
Picking out the proper database is a important part of setting up scalable apps. Not all databases are constructed the same, and utilizing the Improper you can sluggish you down or perhaps result in failures as your application grows.
Start off by comprehending your details. Could it be highly structured, like rows in a very table? If Certainly, a relational database like PostgreSQL or MySQL is a good fit. These are typically robust with interactions, transactions, and consistency. In addition they assist scaling techniques like examine replicas, indexing, and partitioning to handle additional visitors and information.
If the information is a lot more flexible—like consumer exercise logs, solution catalogs, or files—think about a NoSQL solution like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at handling massive volumes of unstructured or semi-structured details and may scale horizontally additional easily.
Also, take into account your read and compose designs. Are you carrying out numerous reads with less writes? Use caching and skim replicas. Are you handling a weighty generate load? Consider databases that could tackle higher compose throughput, or maybe occasion-based mostly facts storage systems like Apache Kafka (for short-term info streams).
It’s also sensible to Imagine in advance. You may not require Superior scaling characteristics now, but choosing a database that supports them indicates you won’t want to change later on.
Use indexing to speed up queries. Keep away from unwanted joins. Normalize or denormalize your details based on your access designs. And constantly watch databases effectiveness when you improve.
Briefly, the appropriate databases will depend on your application’s framework, velocity desires, And just how you assume it to increase. Just take time to choose properly—it’ll save a lot of trouble afterwards.
Improve Code and Queries
Speedy code is essential to scalability. As your app grows, each and every little delay provides up. Inadequately composed code or unoptimized queries can slow down performance and overload your procedure. That’s why it’s essential to Establish successful logic from the start.
Begin by writing clean up, basic code. Stay away from repeating logic and remove just about anything unneeded. Don’t choose the most complicated solution if a simple just one operates. Keep your capabilities quick, focused, and simple to test. Use profiling instruments to discover bottlenecks—places wherever your code will take way too long to operate or utilizes far too much memory.
Following, look at your databases queries. These frequently sluggish issues down in excess of the code itself. Ensure that Just about every query only asks for the info you actually need to have. Avoid Decide on *, which fetches everything, and as a substitute select distinct fields. Use indexes to hurry up lookups. And stay away from accomplishing too many joins, Primarily across massive tables.
If you recognize a similar information currently being asked for again and again, use caching. Retailer the effects temporarily employing applications like Redis or Memcached which means you don’t should repeat expensive operations.
Also, batch your database functions any time you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and can make your application extra efficient.
Make sure to exam with big datasets. Code and queries that perform wonderful with a hundred documents might crash once they have to deal with 1 million.
In a nutshell, scalable apps are rapidly applications. Keep your code tight, your queries lean, and use caching when required. These actions aid your application remain easy and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your app grows, it's to deal with a lot more consumers and a lot more traffic. If everything goes through one server, it will quickly turn into a bottleneck. That’s where by load balancing and caching are available. Both of these instruments support maintain your app quickly, stable, and scalable.
Load balancing spreads incoming website targeted traffic across numerous servers. Rather than one server doing many of the get the job done, the load balancer routes end users to distinct servers according to availability. This means no one server will get overloaded. If a single server goes down, the load balancer can send traffic to the Many others. Instruments like Nginx, HAProxy, or cloud-based mostly options from AWS and Google Cloud make this straightforward to build.
Caching is about storing info temporarily so it might be reused swiftly. When customers ask for precisely the same info all over again—like a product webpage or a profile—you don’t should fetch it from your database whenever. You are able to provide it from your cache.
There's two widespread kinds of caching:
one. Server-side caching (like Redis or Memcached) outlets information in memory for rapid entry.
2. Customer-facet caching (like browser caching or CDN caching) merchants static files near to the person.
Caching decreases databases load, improves pace, and makes your application more productive.
Use caching for things which don’t transform frequently. And generally make certain your cache is up-to-date when data does modify.
To put it briefly, load balancing and caching are straightforward but highly effective tools. Collectively, they assist your app manage additional customers, remain quick, and Recuperate from challenges. If you plan to expand, you require both.
Use Cloud and Container Resources
To create scalable purposes, you need resources that allow your application improve easily. That’s exactly where cloud platforms and containers are available in. They provide you flexibility, decrease setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon World-wide-web Products and services (AWS), Google Cloud System (GCP), and Microsoft Azure let you rent servers and solutions as you will need them. You don’t really need to obtain components or guess upcoming potential. When traffic increases, you are able to incorporate far more methods with just a couple clicks or mechanically working with car-scaling. When website traffic drops, you may scale down to economize.
These platforms also offer you companies like managed databases, storage, load balancing, and protection equipment. It is possible to target constructing your app rather than controlling infrastructure.
Containers are Yet another crucial Instrument. A container offers your application and almost everything it has to run—code, libraries, configurations—into just one device. This makes it easy to maneuver your app in between environments, from a notebook for the cloud, with out surprises. Docker is the most well-liked tool for this.
Once your app utilizes various containers, instruments like Kubernetes enable you to handle them. Kubernetes handles deployment, scaling, and Restoration. If a single part within your app crashes, it restarts it automatically.
Containers also help it become simple to different areas of your app into expert services. It is possible to update or scale components independently, which happens to be great for performance and dependability.
In short, working with cloud and container resources suggests you'll be able to scale speedy, deploy very easily, and Get better swiftly when complications take place. If you prefer your app to improve with out boundaries, start applying these resources early. They help save time, decrease chance, and help you remain centered on setting up, not fixing.
Keep an eye on Everything
Should you don’t watch your software, you won’t know when factors go Completely wrong. Monitoring aids the thing is how your application is performing, place challenges early, and make much better choices as your application grows. It’s a critical part of developing scalable techniques.
Start out by monitoring basic metrics like CPU usage, memory, disk Area, and response time. These let you know how your servers and companies are accomplishing. Applications like Prometheus, Grafana, Datadog, or New Relic can help you gather and visualize this info.
Don’t just check your servers—keep an eye on your application too. Keep an eye on how long it takes for customers to load webpages, how often mistakes take place, and in which they arise. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s taking place inside your code.
Create alerts for crucial difficulties. As an example, Should your response time goes above a Restrict or simply a company goes down, you'll want to get notified instantly. This helps you fix challenges rapid, generally ahead of consumers even discover.
Monitoring is usually handy if you make adjustments. In the event you deploy a new aspect and find out a spike in mistakes or slowdowns, you can roll it again ahead of it leads to real problems.
As your app grows, traffic and facts maximize. With no monitoring, you’ll pass up signs of hassle until eventually it’s as well late. But with the ideal equipment in place, you keep in control.
Briefly, monitoring can help you keep your application dependable and scalable. It’s not pretty much spotting failures—it’s about understanding your technique and making sure it really works well, even under pressure.
Remaining Ideas
Scalability isn’t only for large companies. Even smaller apps need to have a solid foundation. By coming up with cautiously, optimizing correctly, and using the proper applications, you'll be able to Make apps that increase effortlessly with out breaking stressed. Get started tiny, Assume big, and Construct clever.